![]() |
X-CUBE-SPN11 for X-NUCLEO-IHM11M1
|
6Step_Lib.c
Go to the documentation of this file.
48 5) MC_SixStep_Ramp_Motor_calc() -> Calculate the acceleration profile step by step for motor during start-up
49 6) MC_SixStep_NEXT_step()-> Generate the next step number according with the direction (CW or CCW)
88 uint16_t HFBuffer[HFBUFFERSIZE]; /*!< Buffer for Potentiometer Value Filtering at the High-Frequency ADC conversion */
411 SIXSTEP_parameters.BEMF_Tdown_count = 0; /* Reset of the Counter to detect Stop motor condition when a stall condition occurs*/
494 Time_vector_tmp = ((uint64_t) 1000 * (uint64_t)1000 * (uint64_t) MCM_Sqrt(((uint64_t)index_startup_motor * (uint64_t)constant_k)))/632455;
511 SIXSTEP_parameters.prescaler_value = (((SIXSTEP_parameters.SYSCLK_frequency/1000000)*T_single_step_first_value)/65535) - 1;
681 /** @defgroup MC_SixStep_Speed_Val_target_potentiometer MC_SixStep_Speed_Val_target_potentiometer
846 if((SIXSTEP_parameters.speed_fdbk_filtered > (target_speed) || SIXSTEP_parameters.speed_fdbk_filtered < (-target_speed)) && SIXSTEP_parameters.VALIDATION_OK !=TRUE)
852 if(SIXSTEP_parameters.SPEED_VALIDATED == TRUE && SIXSTEP_parameters.BEMF_OK == TRUE && SIXSTEP_parameters.CL_READY != TRUE)
865 SIXSTEP_parameters.Current_Reference = (uint16_t)MC_PI_Controller(&PI_parameters,(int16_t)SIXSTEP_parameters.speed_fdbk_filtered);
869 SIXSTEP_parameters.Current_Reference = (uint16_t)(-MC_PI_Controller(&PI_parameters,(int16_t)SIXSTEP_parameters.speed_fdbk_filtered));
898 if ((SIXSTEP_parameters.Speed_Ref_filtered - SIXSTEP_parameters.Speed_target_ramp) > ADC_SPEED_TH)
909 if ((SIXSTEP_parameters.Speed_target_ramp - SIXSTEP_parameters.Speed_Ref_filtered) > ADC_SPEED_TH)
1030 El_Speed_Hz = (int32_t)((SIXSTEP_parameters.SYSCLK_frequency)/(prsc))/(__HAL_TIM_GetAutoreload(&LF_TIMx)*6);
1119 * @brief Low Frequency Timer Callback - Call the next step and request the filtered speed value
1573 * @brief GPIO EXT Callback - Start or Stop the motor through the Blue push button on STM32Nucleo
void MC_SixStep_DisableInput_CH1_D_CH2_D_CH3_D(void)
Enable Input channel CH2 and CH3 for STSPIN230.
Definition: stm32F401_nucleo_ihm11m1.c:327
void MC_SixStep_Current_Reference_Start(void)
Enable the Current Reference generation.
Definition: stm32F401_nucleo_ihm11m1.c:441
void MC_SixStep_Current_Reference_Setvalue(uint16_t)
Set the value for Current Reference.
Definition: stm32F401_nucleo_ihm11m1.c:480
void MC_SixStep_Stop_PWM_driving(void)
Disable PWM channels for STSPIN230.
Definition: stm32F401_nucleo_ihm11m1.c:363
Definition: 6Step_Lib.h:72
uint64_t MCM_Sqrt(uint64_t)
It calculates the square root of a non-negative s64.
Definition: 6Step_Lib.c:533
void MC_SixStep_EnableInput_CH1_E_CH2_E_CH3_D(void)
Enable Input channel CH1 and CH2 for STSPIN230.
Definition: stm32F401_nucleo_ihm11m1.c:273
void MC_SixStep_EnableInput_CH1_D_CH2_E_CH3_E(void)
Enable Input channel CH2 and CH3 for STSPIN230.
Definition: stm32F401_nucleo_ihm11m1.c:309
void MC_SixStep_Start_PWM_driving(void)
Enable PWM channels for STSPIN230.
Definition: stm32F401_nucleo_ihm11m1.c:345
void UART_Set_Value(void)
void MC_SixStep_HF_TIMx_SetDutyCycle_CH3(uint16_t)
Set the Duty Cycle value for CH3.
Definition: stm32F401_nucleo_ihm11m1.c:423
Definition: 6Step_Lib.h:71
Definition: 6Step_Lib.h:75
void MC_SixStep_Current_Reference_Stop(void)
Disable the Current Reference generation.
Definition: stm32F401_nucleo_ihm11m1.c:460
void MC_SysTick_SixStep_MediumFrequencyTask(void)
Definition: 6Step_Lib.c:1246
int16_t Upper_Limit_Output
Definition: 6Step_Lib.h:176
Definition: 6Step_Lib.h:76
Definition: 6Step_Lib.h:79
void MC_SixStep_HF_TIMx_SetDutyCycle_CH1(uint16_t)
Set the Duty Cycle value for CH1.
Definition: stm32F401_nucleo_ihm11m1.c:381
Definition: 6Step_Lib.h:77
void MC_SixStep_HF_TIMx_SetDutyCycle_CH2(uint16_t)
Set the Duty Cycle value for CH2.
Definition: stm32F401_nucleo_ihm11m1.c:400
Definition: 6Step_Lib.h:73
void SET_DAC_value(uint16_t dac_value)
Set DAC value for debug.
Definition: stm32F401_nucleo_ihm11m1.c:185
uint16_t ADC_BEMF_threshold_UP
Definition: 6Step_Lib.h:113
uint16_t MediumFrequencyTask_flag
Definition: 6Step_Lib.h:142
uint32_t CurrentRegular_BEMF_ch
Definition: 6Step_Lib.h:107
Definition: 6Step_Lib.h:74
This header file provides the set of functions for Motor Control library.
void CMD_Parser(char *pCommandString)
int16_t MC_PI_Controller(SIXSTEP_PI_PARAM_InitTypeDef_t *, int16_t)
Definition: 6Step_Lib.c:768
void MC_SixStep_Speed_Val_target_potentiometer(void)
Definition: 6Step_Lib.c:687
uint16_t ADC_BEMF_threshold_DOWN
Definition: 6Step_Lib.h:114
int16_t Lower_Limit_Output
Definition: 6Step_Lib.h:175
void UART_Communication_Task(void)
void MC_SixStep_EnableInput_CH1_E_CH2_D_CH3_E(void)
Enable Input channel CH1 and CH3 for STSPIN230.
Definition: stm32F401_nucleo_ihm11m1.c:291
void MC_UI_INIT(void)
void MC_SixStep_ADC_Channel(uint32_t adc_ch)
Select the new ADC Channel.
Definition: stm32F401_nucleo_ihm11m1.c:74
Definition: 6Step_Lib.h:80
void HAL_IncTick(void)
This function is called to increment a global variable "uwTick" used as application time base...
Definition: 6Step_Lib.c:1607
uint32_t ADC_Regular_Buffer[5]
Definition: 6Step_Lib.h:112
Generated by
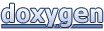