STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_sdram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_sdram.c 00004 * @author MCD Application Team 00005 * @version V2.1.3 00006 * @date 13-January-2016 00007 * @brief This file provides a set of functions needed to drive the 00008 * IS42S16400J SDRAM memory mounted on STM32F429I-Discovery Kit. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32f429i_discovery_sdram.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32F429I_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32F429I_DISCOVERY_SDRAM STM32F429I DISCOVERY SDRAM 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Private_Types_Definitions STM32F429I DISCOVERY SDRAM Private Types Definitions 00055 * @{ 00056 */ 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Private_Defines STM32F429I DISCOVERY SDRAM Private Defines 00062 * @{ 00063 */ 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Private_Macros STM32F429I DISCOVERY SDRAM Private Macros 00069 * @{ 00070 */ 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Private_Variables STM32F429I DISCOVERY SDRAM Private Variables 00076 * @{ 00077 */ 00078 static SDRAM_HandleTypeDef SdramHandle; 00079 static FMC_SDRAM_TimingTypeDef Timing; 00080 static FMC_SDRAM_CommandTypeDef Command; 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Private_Function_Prototypes STM32F429I DISCOVERY SDRAM Private Function Prototypes 00086 * @{ 00087 */ 00088 static void MspInit(void); 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Private_Functions STM32F429I DISCOVERY SDRAM Private Functions 00094 * @{ 00095 */ 00096 00097 /** 00098 * @brief Initializes the SDRAM device. 00099 */ 00100 void BSP_SDRAM_Init(void) 00101 { 00102 /* SDRAM device configuration */ 00103 SdramHandle.Instance = FMC_SDRAM_DEVICE; 00104 00105 /* FMC Configuration -------------------------------------------------------*/ 00106 /* FMC SDRAM Bank configuration */ 00107 /* Timing configuration for 90 Mhz of SD clock frequency (180Mhz/2) */ 00108 /* TMRD: 2 Clock cycles */ 00109 Timing.LoadToActiveDelay = 2; 00110 /* TXSR: min=70ns (7x11.11ns) */ 00111 Timing.ExitSelfRefreshDelay = 7; 00112 /* TRAS: min=42ns (4x11.11ns) max=120k (ns) */ 00113 Timing.SelfRefreshTime = 4; 00114 /* TRC: min=70 (7x11.11ns) */ 00115 Timing.RowCycleDelay = 7; 00116 /* TWR: min=1+ 7ns (1+1x11.11ns) */ 00117 Timing.WriteRecoveryTime = 2; 00118 /* TRP: 20ns => 2x11.11ns*/ 00119 Timing.RPDelay = 2; 00120 /* TRCD: 20ns => 2x11.11ns */ 00121 Timing.RCDDelay = 2; 00122 00123 /* FMC SDRAM control configuration */ 00124 SdramHandle.Init.SDBank = FMC_SDRAM_BANK2; 00125 /* Row addressing: [7:0] */ 00126 SdramHandle.Init.ColumnBitsNumber = FMC_SDRAM_COLUMN_BITS_NUM_8; 00127 /* Column addressing: [11:0] */ 00128 SdramHandle.Init.RowBitsNumber = FMC_SDRAM_ROW_BITS_NUM_12; 00129 SdramHandle.Init.MemoryDataWidth = SDRAM_MEMORY_WIDTH; 00130 SdramHandle.Init.InternalBankNumber = FMC_SDRAM_INTERN_BANKS_NUM_4; 00131 SdramHandle.Init.CASLatency = SDRAM_CAS_LATENCY; 00132 SdramHandle.Init.WriteProtection = FMC_SDRAM_WRITE_PROTECTION_DISABLE; 00133 SdramHandle.Init.SDClockPeriod = SDCLOCK_PERIOD; 00134 SdramHandle.Init.ReadBurst = SDRAM_READBURST; 00135 SdramHandle.Init.ReadPipeDelay = FMC_SDRAM_RPIPE_DELAY_1; 00136 00137 /* SDRAM controller initialization */ 00138 MspInit(); 00139 HAL_SDRAM_Init(&SdramHandle, &Timing); 00140 00141 /* SDRAM initialization sequence */ 00142 BSP_SDRAM_Initialization_sequence(REFRESH_COUNT); 00143 } 00144 00145 /** 00146 * @brief Programs the SDRAM device. 00147 * @param RefreshCount: SDRAM refresh counter value 00148 */ 00149 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount) 00150 { 00151 __IO uint32_t tmpmrd =0; 00152 00153 /* Step 1: Configure a clock configuration enable command */ 00154 Command.CommandMode = FMC_SDRAM_CMD_CLK_ENABLE; 00155 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00156 Command.AutoRefreshNumber = 1; 00157 Command.ModeRegisterDefinition = 0; 00158 00159 /* Send the command */ 00160 HAL_SDRAM_SendCommand(&SdramHandle, &Command, SDRAM_TIMEOUT); 00161 00162 /* Step 2: Insert 100 us minimum delay */ 00163 /* Inserted delay is equal to 1 ms due to systick time base unit (ms) */ 00164 HAL_Delay(1); 00165 00166 /* Step 3: Configure a PALL (precharge all) command */ 00167 Command.CommandMode = FMC_SDRAM_CMD_PALL; 00168 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00169 Command.AutoRefreshNumber = 1; 00170 Command.ModeRegisterDefinition = 0; 00171 00172 /* Send the command */ 00173 HAL_SDRAM_SendCommand(&SdramHandle, &Command, SDRAM_TIMEOUT); 00174 00175 /* Step 4: Configure an Auto Refresh command */ 00176 Command.CommandMode = FMC_SDRAM_CMD_AUTOREFRESH_MODE; 00177 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00178 Command.AutoRefreshNumber = 4; 00179 Command.ModeRegisterDefinition = 0; 00180 00181 /* Send the command */ 00182 HAL_SDRAM_SendCommand(&SdramHandle, &Command, SDRAM_TIMEOUT); 00183 00184 /* Step 5: Program the external memory mode register */ 00185 tmpmrd = (uint32_t)SDRAM_MODEREG_BURST_LENGTH_1 | 00186 SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL | 00187 SDRAM_MODEREG_CAS_LATENCY_3 | 00188 SDRAM_MODEREG_OPERATING_MODE_STANDARD | 00189 SDRAM_MODEREG_WRITEBURST_MODE_SINGLE; 00190 00191 Command.CommandMode = FMC_SDRAM_CMD_LOAD_MODE; 00192 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00193 Command.AutoRefreshNumber = 1; 00194 Command.ModeRegisterDefinition = tmpmrd; 00195 00196 /* Send the command */ 00197 HAL_SDRAM_SendCommand(&SdramHandle, &Command, SDRAM_TIMEOUT); 00198 00199 /* Step 6: Set the refresh rate counter */ 00200 /* Set the device refresh rate */ 00201 HAL_SDRAM_ProgramRefreshRate(&SdramHandle, RefreshCount); 00202 } 00203 00204 /** 00205 * @brief Reads an mount of data from the SDRAM memory in polling mode. 00206 * @param uwStartAddress : Read start address 00207 * @param pData : Pointer to data to be read 00208 * @param uwDataSize: Size of read data from the memory 00209 */ 00210 void BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00211 { 00212 HAL_SDRAM_Read_32b(&SdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize); 00213 } 00214 00215 /** 00216 * @brief Reads an mount of data from the SDRAM memory in DMA mode. 00217 * @param uwStartAddress : Read start address 00218 * @param pData : Pointer to data to be read 00219 * @param uwDataSize: Size of read data from the memory 00220 */ 00221 void BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00222 { 00223 HAL_SDRAM_Read_DMA(&SdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize); 00224 } 00225 00226 /** 00227 * @brief Writes an mount of data to the SDRAM memory in polling mode. 00228 * @param uwStartAddress : Write start address 00229 * @param pData : Pointer to data to be written 00230 * @param uwDataSize: Size of written data from the memory 00231 */ 00232 void BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00233 { 00234 /* Disable write protection */ 00235 HAL_SDRAM_WriteProtection_Disable(&SdramHandle); 00236 00237 /*Write 32-bit data buffer to SDRAM memory*/ 00238 HAL_SDRAM_Write_32b(&SdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize); 00239 } 00240 00241 /** 00242 * @brief Writes an mount of data to the SDRAM memory in DMA mode. 00243 * @param uwStartAddress : Write start address 00244 * @param pData : Pointer to data to be written 00245 * @param uwDataSize: Size of written data from the memory 00246 */ 00247 void BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00248 { 00249 HAL_SDRAM_Write_DMA(&SdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize); 00250 } 00251 00252 /** 00253 * @brief Sends command to the SDRAM bank. 00254 * @param SdramCmd: Pointer to SDRAM command structure 00255 * @retval HAL status 00256 */ 00257 HAL_StatusTypeDef BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd) 00258 { 00259 return(HAL_SDRAM_SendCommand(&SdramHandle, SdramCmd, SDRAM_TIMEOUT)); 00260 } 00261 00262 /** 00263 * @brief Handles SDRAM DMA transfer interrupt request. 00264 */ 00265 void BSP_SDRAM_DMA_IRQHandler(void) 00266 { 00267 HAL_DMA_IRQHandler(SdramHandle.hdma); 00268 } 00269 00270 /** 00271 * @brief Initializes SDRAM MSP. 00272 */ 00273 static void MspInit(void) 00274 { 00275 static DMA_HandleTypeDef dmaHandle; 00276 GPIO_InitTypeDef GPIO_InitStructure; 00277 SDRAM_HandleTypeDef *hsdram = &SdramHandle; 00278 00279 /* Enable FMC clock */ 00280 __FMC_CLK_ENABLE(); 00281 00282 /* Enable chosen DMAx clock */ 00283 __DMAx_CLK_ENABLE(); 00284 00285 /* Enable GPIOs clock */ 00286 __GPIOB_CLK_ENABLE(); 00287 __GPIOC_CLK_ENABLE(); 00288 __GPIOD_CLK_ENABLE(); 00289 __GPIOE_CLK_ENABLE(); 00290 __GPIOF_CLK_ENABLE(); 00291 __GPIOG_CLK_ENABLE(); 00292 00293 /*-- GPIOs Configuration -----------------------------------------------------*/ 00294 /* 00295 +-------------------+--------------------+--------------------+--------------------+ 00296 + SDRAM pins assignment + 00297 +-------------------+--------------------+--------------------+--------------------+ 00298 | PD0 <-> FMC_D2 | PE0 <-> FMC_NBL0 | PF0 <-> FMC_A0 | PG0 <-> FMC_A10 | 00299 | PD1 <-> FMC_D3 | PE1 <-> FMC_NBL1 | PF1 <-> FMC_A1 | PG1 <-> FMC_A11 | 00300 | PD8 <-> FMC_D13 | PE7 <-> FMC_D4 | PF2 <-> FMC_A2 | PG8 <-> FMC_SDCLK | 00301 | PD9 <-> FMC_D14 | PE8 <-> FMC_D5 | PF3 <-> FMC_A3 | PG15 <-> FMC_NCAS | 00302 | PD10 <-> FMC_D15 | PE9 <-> FMC_D6 | PF4 <-> FMC_A4 |--------------------+ 00303 | PD14 <-> FMC_D0 | PE10 <-> FMC_D7 | PF5 <-> FMC_A5 | 00304 | PD15 <-> FMC_D1 | PE11 <-> FMC_D8 | PF11 <-> FMC_NRAS | 00305 +-------------------| PE12 <-> FMC_D9 | PF12 <-> FMC_A6 | 00306 | PE13 <-> FMC_D10 | PF13 <-> FMC_A7 | 00307 | PE14 <-> FMC_D11 | PF14 <-> FMC_A8 | 00308 | PE15 <-> FMC_D12 | PF15 <-> FMC_A9 | 00309 +-------------------+--------------------+--------------------+ 00310 | PB5 <-> FMC_SDCKE1| 00311 | PB6 <-> FMC_SDNE1 | 00312 | PC0 <-> FMC_SDNWE | 00313 +-------------------+ 00314 00315 */ 00316 00317 /* Common GPIO configuration */ 00318 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00319 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00320 GPIO_InitStructure.Pull = GPIO_NOPULL; 00321 GPIO_InitStructure.Alternate = GPIO_AF12_FMC; 00322 00323 /* GPIOB configuration */ 00324 GPIO_InitStructure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00325 HAL_GPIO_Init(GPIOB, &GPIO_InitStructure); 00326 00327 /* GPIOC configuration */ 00328 GPIO_InitStructure.Pin = GPIO_PIN_0; 00329 HAL_GPIO_Init(GPIOC, &GPIO_InitStructure); 00330 00331 /* GPIOD configuration */ 00332 GPIO_InitStructure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | 00333 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | 00334 GPIO_PIN_15; 00335 HAL_GPIO_Init(GPIOD, &GPIO_InitStructure); 00336 00337 /* GPIOE configuration */ 00338 GPIO_InitStructure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | 00339 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00340 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00341 GPIO_PIN_14 | GPIO_PIN_15; 00342 HAL_GPIO_Init(GPIOE, &GPIO_InitStructure); 00343 00344 /* GPIOF configuration */ 00345 GPIO_InitStructure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00346 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | 00347 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00348 GPIO_PIN_14 | GPIO_PIN_15; 00349 HAL_GPIO_Init(GPIOF, &GPIO_InitStructure); 00350 00351 /* GPIOG configuration */ 00352 GPIO_InitStructure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | 00353 GPIO_PIN_5 | GPIO_PIN_8 | GPIO_PIN_15; 00354 HAL_GPIO_Init(GPIOG, &GPIO_InitStructure); 00355 00356 /* Configure common DMA parameters */ 00357 dmaHandle.Init.Channel = SDRAM_DMAx_CHANNEL; 00358 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00359 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00360 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00361 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00362 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00363 dmaHandle.Init.Mode = DMA_NORMAL; 00364 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00365 dmaHandle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00366 dmaHandle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00367 dmaHandle.Init.MemBurst = DMA_MBURST_SINGLE; 00368 dmaHandle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00369 00370 dmaHandle.Instance = SDRAM_DMAx_STREAM; 00371 00372 /* Associate the DMA handle */ 00373 __HAL_LINKDMA(hsdram, hdma, dmaHandle); 00374 00375 /* Deinitialize the stream for new transfer */ 00376 HAL_DMA_DeInit(&dmaHandle); 00377 00378 /* Configure the DMA stream */ 00379 HAL_DMA_Init(&dmaHandle); 00380 00381 /* NVIC configuration for DMA transfer complete interrupt */ 00382 HAL_NVIC_SetPriority(SDRAM_DMAx_IRQn, 0, 0); 00383 HAL_NVIC_EnableIRQ(SDRAM_DMAx_IRQn); 00384 } 00385 00386 /** 00387 * @} 00388 */ 00389 00390 /** 00391 * @} 00392 */ 00393 00394 /** 00395 * @} 00396 */ 00397 00398 /** 00399 * @} 00400 */ 00401 00402 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 13:22:14 for STM32F429I-Discovery BSP User Manual by
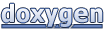