STM32446E_EVAL BSP User Manual
|
stm32446e_eval_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval_ts.c 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file provides a set of functions needed to manage the Touch 00008 * Screen on STM32446E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM32446E-EVAL 00044 evaluation board on AMPIRE 480x272 LCD mounted on MB1046 daughter board. 00045 - the MFXSTM32L152 IO expander device component 00046 driver must be included in order to run the TS module commanded by the IO 00047 expander device, the MFX IO expander device component driver must be 00048 also included in case of interrupt mode use of the TS. 00049 00050 2. Driver description: 00051 --------------------- 00052 + Initialization steps: 00053 o Initialize the TS module using the BSP_TS_Init() function. This 00054 function includes the MSP layer hardware resources initialization and the 00055 communication layer configuration to start the TS use. The LCD size properties 00056 (x and y) are passed as parameters. 00057 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00058 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00059 as an external interrupt whenever a touch is detected. 00060 The interrupt mode internally uses the IO functionalities driver driven by 00061 the IO expander, to configure the IT line. 00062 00063 + Touch screen use 00064 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00065 used. This function returns information about the last LCD touch occurred 00066 in the TS_StateTypeDef structure. 00067 o If TS interrupt mode is used, the function BSP_TS_ITGetStatus() is needed to get 00068 the interrupt status. To clear the IT pending bits, you should call the 00069 function BSP_TS_ITClear(). 00070 o The IT is handled using the corresponding external interrupt IRQ handler, 00071 the user IT callback treatment is implemented on the same external interrupt 00072 callback. 00073 00074 ------------------------------------------------------------------------------*/ 00075 00076 /* Includes ------------------------------------------------------------------*/ 00077 #include "stm32446e_eval_ts.h" 00078 #include "stm32446e_eval_io.h" 00079 00080 /** @addtogroup BSP 00081 * @{ 00082 */ 00083 00084 /** @addtogroup STM32446E_EVAL 00085 * @{ 00086 */ 00087 00088 /** @defgroup STM32446E_EVAL_TS STM32446E EVAL TS 00089 * @{ 00090 */ 00091 00092 /** @defgroup STM32446E_EVAL_TS_Private_Types_Definitions STM32446E EVAL TS Private Types Definitions 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32446E_EVAL_TS_Private_Defines STM32446E EVAL TS Private Defines 00100 * @{ 00101 */ 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32446E_EVAL_TS_Private_Macros STM32446E EVAL TS Private Macros 00107 * @{ 00108 */ 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32446E_EVAL_TS_Private_Variables STM32446E EVAL TS Private Variables 00114 * @{ 00115 */ 00116 static TS_DrvTypeDef *ts_driver; 00117 static uint16_t tsBundaryX, tsBundaryY; 00118 static uint8_t tsOrientation; 00119 static uint8_t AddressI2C; 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32446E_EVAL_TS_Private_Function_Prototypes STM32446E EVAL TS Private Function Prototypes 00125 * @{ 00126 */ 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM32446E_EVAL_TS_Private_Functions STM32446E EVAL TS Private Functions 00132 * @{ 00133 */ 00134 00135 /** 00136 * @brief Initializes and configures the touch screen functionalities and 00137 * configures all necessary hardware resources (GPIOs, clocks..). 00138 * @param xSize: Maximum X size of the TS area on LCD 00139 * @param ySize: Maximum Y size of the TS area on LCD 00140 * @retval TS_OK if all initializations are OK. Other value if error. 00141 */ 00142 uint8_t BSP_TS_Init(uint16_t xSize, uint16_t ySize) 00143 { 00144 uint8_t mfxstm32l152_id = 0; 00145 00146 tsBundaryX = xSize; 00147 tsBundaryY = ySize; 00148 00149 /* Initialize IO functionalities (MFX) used by TS */ 00150 BSP_IO_Init(); 00151 00152 /* Read ID and verify if the IO expander is ready */ 00153 mfxstm32l152_id = mfxstm32l152_io_drv.ReadID(IO_I2C_ADDRESS); 00154 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00155 { 00156 /* Initialize the TS driver structure */ 00157 ts_driver = &mfxstm32l152_ts_drv; 00158 AddressI2C = TS_I2C_ADDRESS; 00159 tsOrientation = TS_SWAP_NONE; 00160 } 00161 00162 00163 /* Initialize the TS driver */ 00164 ts_driver->Init(AddressI2C); 00165 ts_driver->Start(AddressI2C); 00166 00167 return TS_OK; 00168 } 00169 00170 /** 00171 * @brief DeInitializes the TouchScreen. 00172 * @retval TS state 00173 */ 00174 uint8_t BSP_TS_DeInit(void) 00175 { 00176 /* Actually ts_driver does not provide a DeInit function */ 00177 return TS_OK; 00178 } 00179 00180 /** 00181 * @brief Enables the touch screen interrupts. 00182 * @retval TS_OK if all initializations are OK. Other value if error. 00183 */ 00184 uint8_t BSP_TS_ITEnable(void) 00185 { 00186 /* Enable the TS ITs */ 00187 ts_driver->EnableIT(AddressI2C); 00188 return TS_OK; 00189 } 00190 00191 /** 00192 * @brief Disables the touch screen interrupts. 00193 * @retval TS_OK if all initializations are OK. Other value if error. 00194 */ 00195 uint8_t BSP_TS_ITDisable(void) 00196 { 00197 /* Disable the TS ITs */ 00198 ts_driver->DisableIT(AddressI2C); 00199 return TS_OK; 00200 } 00201 00202 /** 00203 * @brief Configures and enables the touch screen interrupts. 00204 * @retval TS_OK if all initializations are OK. Other value if error. 00205 */ 00206 uint8_t BSP_TS_ITConfig(void) 00207 { 00208 /* Initialize the IO */ 00209 BSP_IO_Init(); 00210 00211 /* Enable the TS ITs */ 00212 ts_driver->EnableIT(AddressI2C); 00213 00214 return TS_OK; 00215 } 00216 00217 /** 00218 * @brief Clears all touch screen interrupts. 00219 */ 00220 void BSP_TS_ITClear(void) 00221 { 00222 /* Clear TS IT pending bits */ 00223 ts_driver->ClearIT(AddressI2C); 00224 } 00225 00226 /** 00227 * @brief Gets the touch screen interrupt status. 00228 * @retval TS_OK if all initializations are OK. Other value if error. 00229 */ 00230 uint8_t BSP_TS_ITGetStatus(void) 00231 { 00232 /* Return the TS IT status */ 00233 return (ts_driver->GetITStatus(AddressI2C)); 00234 } 00235 00236 /** 00237 * @brief Clears touch screen FIFO containing 128 x,y values. 00238 */ 00239 void BSP_TS_FIFOClear(void) 00240 { 00241 /* ts.h does not foresee an API that allows clearing the FIFO */ 00242 /* reading GetXY currently read one value from the FIFO */ 00243 /* but the FIFO can contain up to 128 values ... */ 00244 /* which would mean 128 I2C read. */ 00245 /* Best is to modify ts.h (not done for compatibility with other families) */ 00246 } 00247 00248 /** 00249 * @brief Returns status and positions of the touch screen. 00250 * @param TS_State: Pointer to touch screen current state structure 00251 * @retval TS_OK if all initializations are OK. Other value if error. 00252 */ 00253 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00254 { 00255 static uint32_t _x = 0, _y = 0; 00256 uint16_t x_diff, y_diff , x , y; 00257 uint16_t swap; 00258 00259 TS_State->TouchDetected = ts_driver->DetectTouch(AddressI2C); 00260 00261 if(TS_State->TouchDetected) 00262 { 00263 ts_driver->GetXY(AddressI2C, &x, &y); 00264 00265 if(tsOrientation & TS_SWAP_X) 00266 { 00267 x = 4096 - x; 00268 } 00269 00270 if(tsOrientation & TS_SWAP_Y) 00271 { 00272 y = 4096 - y; 00273 } 00274 00275 if(tsOrientation & TS_SWAP_XY) 00276 { 00277 swap = y; 00278 y = x; 00279 x = swap; 00280 } 00281 00282 x_diff = x > _x? (x - _x): (_x - x); 00283 y_diff = y > _y? (y - _y): (_y - y); 00284 00285 if (x_diff + y_diff > 5) 00286 { 00287 _x = x; 00288 _y = y; 00289 } 00290 00291 TS_State->x = (tsBundaryX * _x) >> 12; 00292 TS_State->y = (tsBundaryY * _y) >> 12; 00293 } 00294 return TS_OK; 00295 } 00296 00297 00298 00299 /** 00300 * @} 00301 */ 00302 00303 /** 00304 * @} 00305 */ 00306 00307 /** 00308 * @} 00309 */ 00310 00311 /** 00312 * @} 00313 */ 00314 00315 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
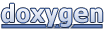